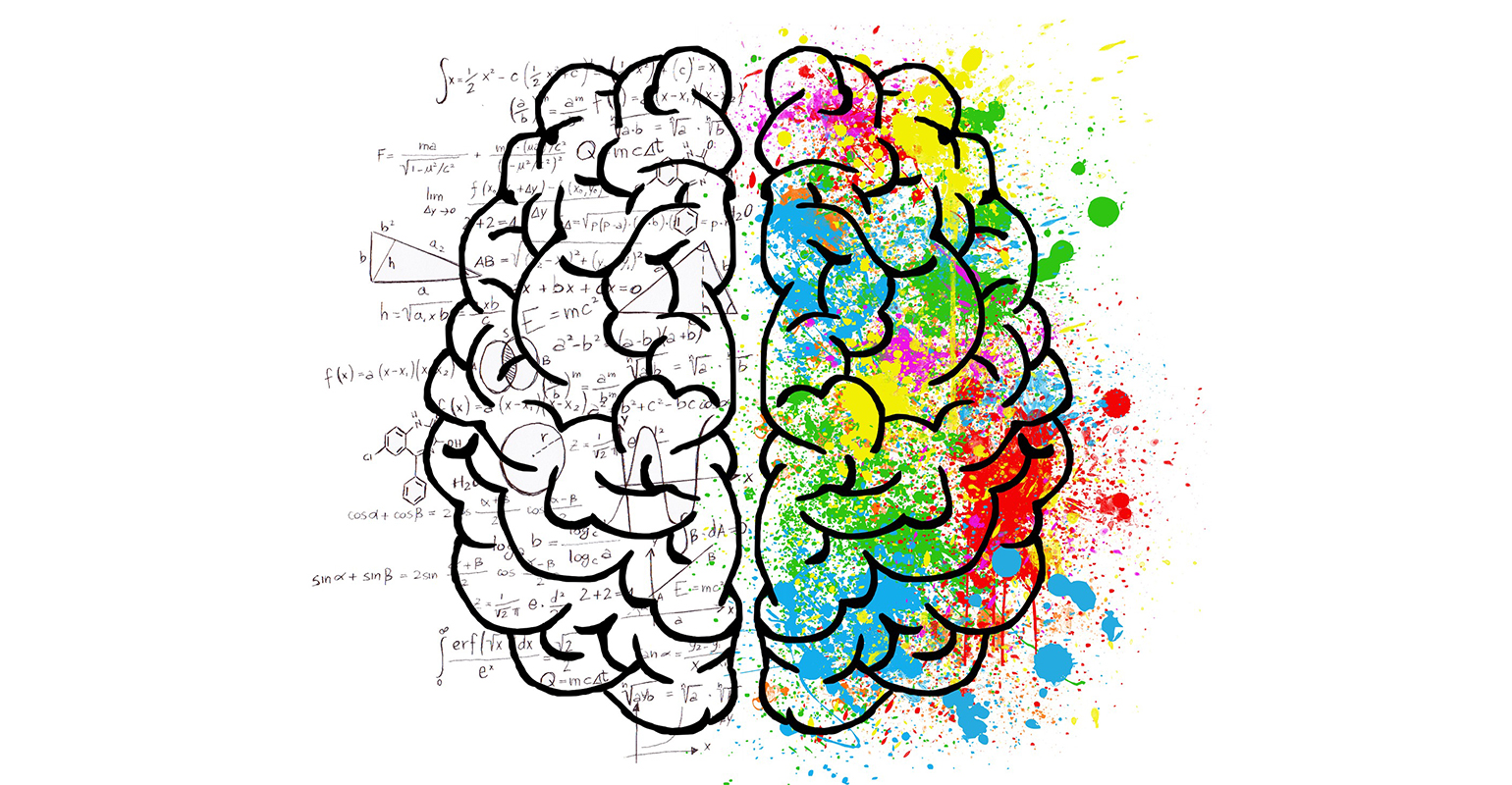
Ages ago I was quite busy scripting in the KornShell. And I had the habit of building quick and dirty graphical interfaces to show the results of the scripts. What I wanted was to look at the IPv6 environment by means of a graphical interface using the Powershell.
So the first thing I did was building some functions in the Powershell (v1.0). Simple graphical functions to be incorporated in my own shell environment, by using the ". " command. That was quite easy because of some excellent blogs.
The functions are written in the Form_Functions_v1.ps1 file.
#
# simple graphical functions
# use the . command to incorporate the functions in the shell environment
#
############################################################################################
[System.Reflection.Assembly]::LoadWithPartialName("System.Drawing")
[System.Reflection.Assembly]::LoadWithPartialName("System.windows.forms")
function CreateForm {
param([string]$t,[int]$h,[int]$w)
$global:Form = new-object System.Windows.Forms.form
$Form.Text = $t
$Form.size = new-object System.Drawing.Size($h,$w)
}
function CreateLabel {
param([string]$t,[int]$x, [int]$y, [int]$w, [int]$h)
$global:Label = new-object System.Windows.Forms.Label
$Label.Text = $t
$Label.Location = new-object System.Drawing.Size($x,$y)
$Label.size = new-object System.Drawing.Size($w,$h)
$Form.Controls.Add($Label)
$label
}
function CreateButton {
param([string]$t,[int]$x,[int]$y,[int]$w, [int]$h)
$global:Button = new-object System.Windows.Forms.Button
$Button.Text = $t
$Button.Location = new-object System.Drawing.Size($x,$y)
$Button.size = new-object System.Drawing.Size($w,$h)
$Form.Controls.Add($Button)
$Button
}
function CreateTextBox {
param([string]$t,[int]$x,[int]$y,[int]$w,[int]$h)
$global:TextBox = new-object System.Windows.Forms.TextBox
$TextBox.Text = $t
$TextBox.Location = new-object System.Drawing.Size($x,$y)
$TextBox.size = new-object System.Drawing.Size($w,$h)
$Form.Controls.Add($TextBox)
$TextBox
}
function CreateListBox {
param([int]$x,[int]$y,[int]$w,[int]$h)
$global:ListBox = new-object System.Windows.Forms.ListBox
$ListBox.Location = new-object System.Drawing.Size($x,$y)
$ListBox.size = new-object System.Drawing.Size($w,$h)
$Form.Controls.Add($ListBox)
$ListBox
}
function CreateComboBox {
param([int]$x,[int]$y)
$global:ComboBox = new-object System.Windows.Forms.ComboBox
$ComboBox.Location = new-object System.Drawing.Size($x,$y)
$ComboBox.size = new-object System.Drawing.Size(200,80)
$Form.Controls.Add($ComboBox)
$ComboBox
}
function ShowForm {
$Form.topmost = $true
$Form.showdialog()
}
I got already used to the Netsh command to investigate the IPv6 environment. But I wanted to look at the netsh interfaces from within the Powershell.
The Powershell works with objects, so the netsh output had to be converted to Powershell objects. Happily there is a New-Object command to create Powershell objects from text-output. I created objects ($MyInterface) by using the Netsh interface ipv6 show interfaces command and placed those objects in an collection of objects ($MyInterfaces) , so I could easily work with them in the scripting environment. The objects from the netsh interface are created in the NetshInterface_v1.ps1 file.
#
# Create an (Array of) Objects from : "netsh ipv6 interface show interfaces"
#
#
############################################################################################
#
# Create an Object
# by parsing the text output from the netsh command
# define properties on the new Object
# assign values to those porterties
# put this object in an array
# this array has a global scope,
# so can be used in the Powershell console after running this file
#
#
############################################################################################ Main
$global:MyInterfaces = @()
$interface = netsh interface ipv6 show interfaces
$property=[regex]::replace($interface[1],'[ ]+'," ").Split(" ")
for ( $i = 3 ; $i -lt 5 ;$i++ ) {
$MyInterface = New-Object PSObject
$content = [regex]::replace($interface[$i],'[ ]+'," ").Split(" ")
for ( $j = 0 ; $j -lt 5 ; $j++ ) {
$MyInterface | Add-Member NoteProperty $property[$j] $content[$j+1]
}
$global:MyInterfaces = $MyInterfaces + @($MyInterface)
}
Next the graphical interface. I wanted to build some interface to quickly inspect the IPV6-interfaces. The idea was to build a drop-down list to gather the the objects to be investigated and to show the attributes and their values in a very simple window. The contents of the windows had to be derived from the object-properties. The enumeration of the created objects and the display are written in the NetshInterfaces_GU_v1.ps1 file.
#
# Simple window. Shows the ipv6 interfaces on the local engine.
# from : "netsh interface ipv6 show interfaces"
#
############################################################################################
#
# In this example script only the IPV6 interfaces
#
# Get the simple graphical functions
# Get the Ipv6 interfaces by calling the NetshInterfaces script.
# this scripts delivers the $MyInterfaces collection
# Create the Form
# Create the Listbox and specify a user action.
# Create $ListOfNames ( properties of the object )
# Create ( an array of ) Labels
# Create ( an array of ) Textboxes
# Show the IPv6 interfaces in the Listbox
# If the user selects an Interface, show the properties of that interface
#
############################################################################################
Function Create_ListOfNames {
param($object)
$ll = @()
$list = $MyInterfaces | gm -type NoteProperty
foreach ( $line in $list ) {$ll = $ll + @($line.name )}
return $ll
}
Function Create_Labels {
param($list, $x, $y, $w, $h )
$ll = @()
for ( $i = 0 ; $i -lt $list.count ; $i++ ) {
$ll = $ll + @(Createlabel $list[$i] $x ($y = $y + 20) $w $h )
}
return $ll
}
Function Create_TextBoxes {
param($list, $x, $y, $w, $h)
$tt = @()
for ( $i = 0 ; $i -lt $list.count ; $i++ ) {
$tt = $tt + @(Createtextbox "" $x ($y = $y + 20) $w $h )
}
return $tt
}
Function ShowListBox_Interfaces {
$ListBox_Interfaces.Items.Clear()
for ( $i = 0 ; $i -lt $MyInterFaces.count ; $i++ ) {
$ListBox_Interfaces.Items.Add($MyInterFaces[$i].Name)
}
}
Function Get_Content_TextBoxes {
$global:thisobject = $MyinterFaces | where { $_.Name -match $ListBox_Interfaces.selectedItem }
$content = $thisobject | gm -type Noteproperty
for ( $i = 0 ; $i -lt $content.count ; $i++ ) {
$Textboxes[$i].Text = $thisobject.($content[$i].Name)
}
}
############################################################################################ Main
. ./Function_Forms_v1.ps1
./NetshInterfaces_v1.ps1
CreateForm "Netsh IPv6 Interfaces" 400 300
$global:ListBox_Interfaces = CreateListBox 60 20 200 60
$ListBox_Interfaces.add_click({ Get_Content_TextBoxes })
$global:ListOfNames = Create_ListOfNames
$global:Labels = Create_Labels $listOfNames 20 120 50 20
$global:Textboxes = Create_TextBoxes $listOfNames 120 120 100 20
ShowListBox_Interfaces
ShowForm
Of course all the syntax and so, are quite crude and may not be as sophisticated as could be. But i'm just starting my investigations, so – in time- maybe you will see some improvements. You can find the Powershell Scripts in the NetshInterface_v1.zip file in the download section.