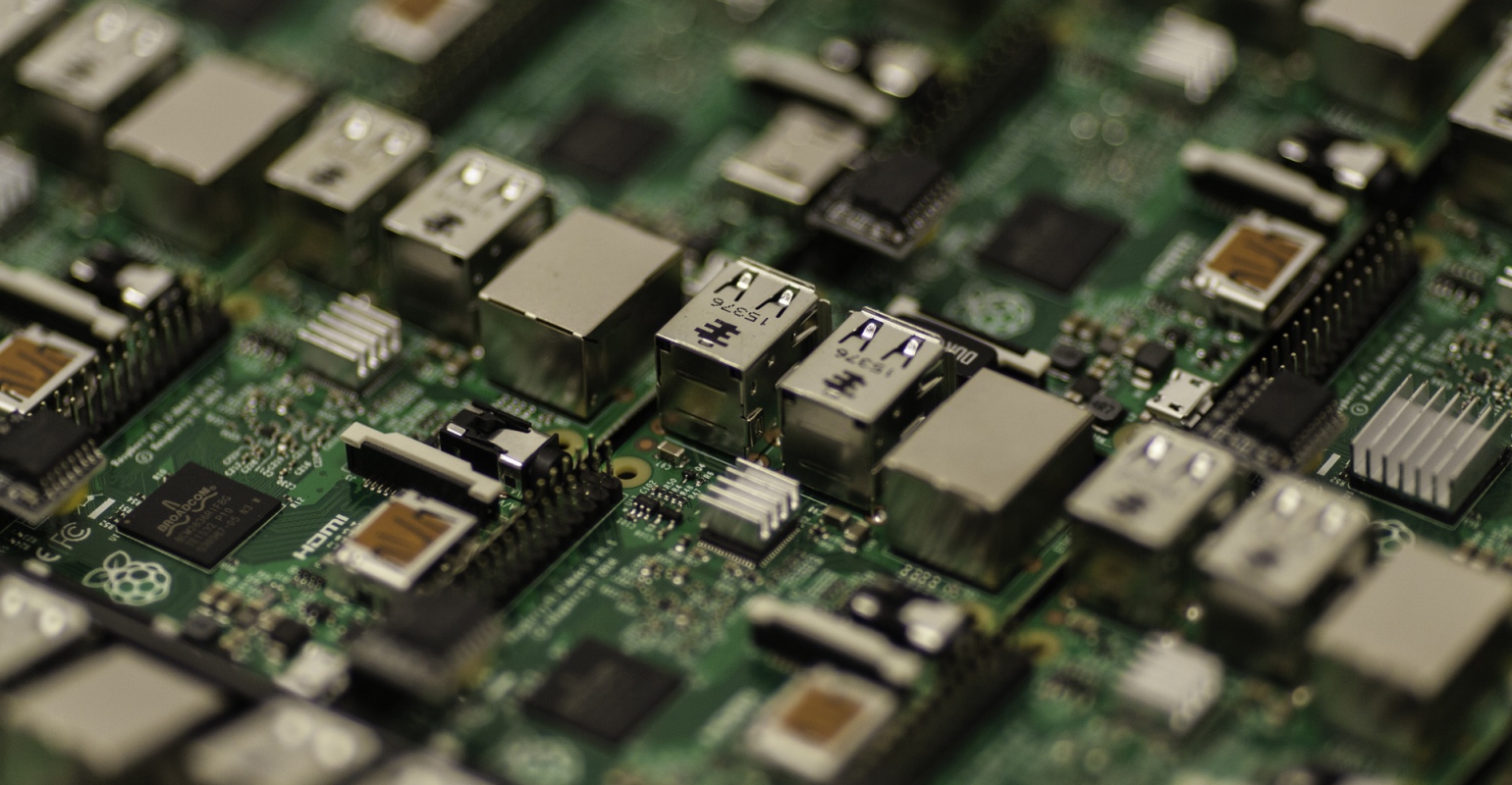
public class TesterForm : System.Windows.Forms.Form}
{
private enum WindowsMessage
{
Paint = 0x000F
}private GameEngine _gameEngine;
public void Initialize(GameEngine gameEngine)
{
#if WINDOWED
this.Size = new Size(640,480);
#else
this.FormBorderStyle = FormBorderStyle.None;
this.Size = Screen.PrimaryScreen.Bounds.Size;
#endif
this.StartPosition = FormStartPosition.CenterScreen;
this.SetStyle(ControlStyles.AllPaintingInWmPaint | ControlStyles.Opaque, true);
_gameEngine = gameEngine;
_gameEngine.Initialize(this);
}private void Frame()
{
if(_gameEngine != null)
{
_gameEngine.ReadInput();
_gameEngine.ProcessGamelogic();
_gameEngine.Render();
}
}protected override void WndProc(ref Message m)
{
switch (m.Msg)
{
case (int)WindowsMessage.Paint:
{
Frame();
break;
}
default:
{
base.WndProc(ref m);
break;
}
}
}
}
As you can see there is no Main in this class. It is my GameEngine class (or a sub class of the GameEngine class) that contains the Main and thus is the entrypoint of the application. The GameEngine class passes an instance of itself to the Form. I think that this is a better design.