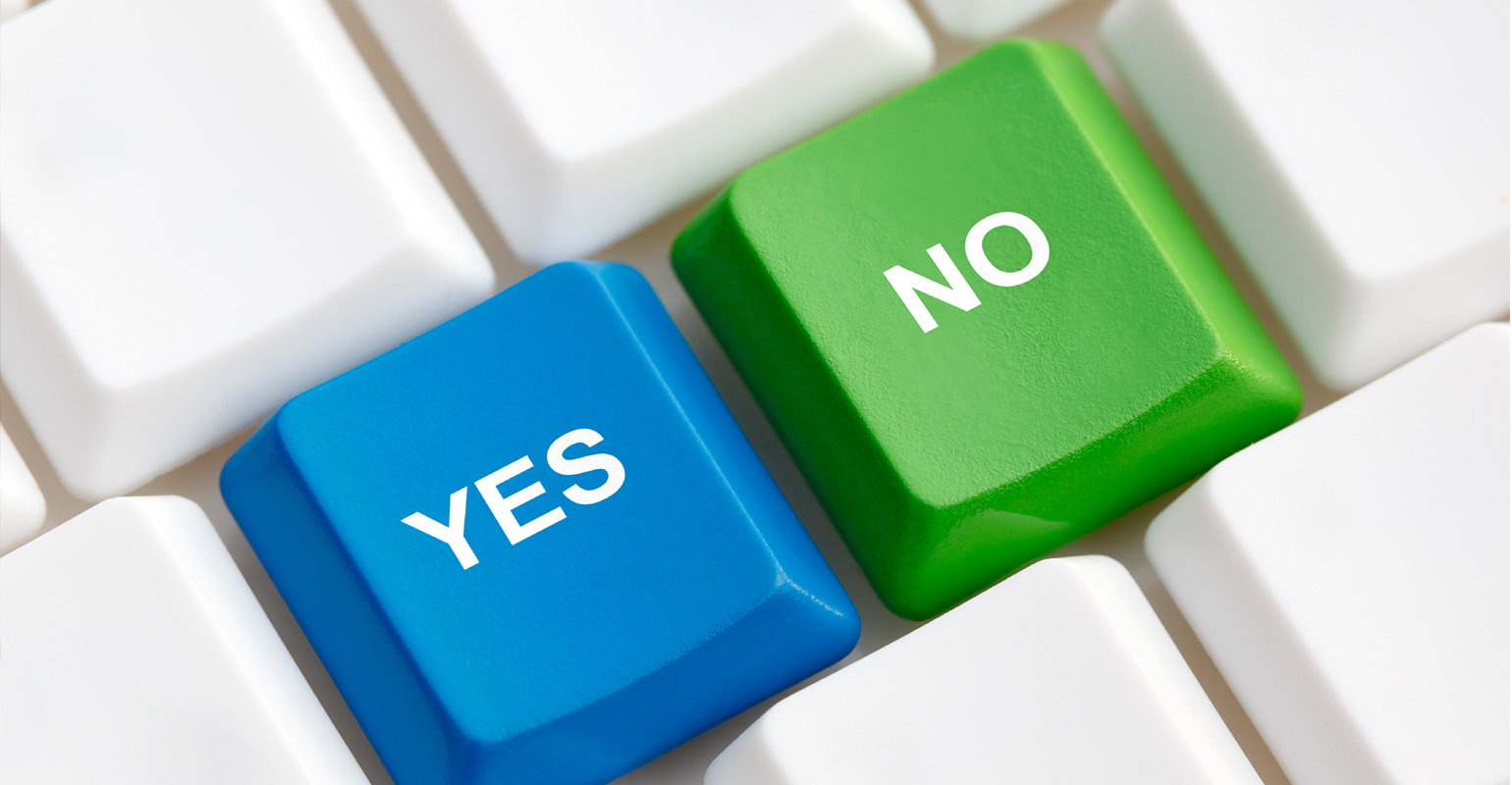
C# has LINQ, but java doesn’t have a true competitor for this. I heard about ideas on building a similar construction for Java, but I don’t think the implementation would be quite the same as in C#. The whole idea of having LINQ for java kept me awake last night, I couldn’t stop thinking about it until I found a simple solution that I could implement today.
I was right when I thought it would be simple, I implemented some simple operators in under 10 minutes without having to break the language anywhere. It sure looks ugly, but LINQ queries can get ugly pretty quickly too if you don’t use extension methods. Here’s a sample query I created using my own implementation:
Iterable<String> subset = Query.select(Query.Where(people, new Predicate<Person>() {
public
boolean evaluate(Person item) { return item.name.equalsIgnoreCase(“David”); } }),
new Selector<Person,String>() { public String select(Person item) { return item.name; } });
It looks cryptic and I wouldn’t want to be the one that has to maintain this code, however it does the job and proves its actually possible to create a simple LINQ implementation in Java. There are some key points that would make this code look better though, one of them is extension methods and the other is delegates.
The whole idea is to create a helper class the implements the operators. Each operator should accept a generic list of items that can be iterated through by the query operator and a predicate, selector or some other kind of helper object that can be used to filter items, convert items or do some other stuff to the input. The where operator is shown as a sample below:
/**
*
Filters
a
list
of
items
by
a
predicate
*
@param
<E> Element
type
to
filter
*
@param
items Items
to
filter
*
@param
predicate Predicate
to
evaluate
for
each
element
*
@return Returns
an
enumeration
of
the
filtered
types
*/
public
static <E> Iterable<E> where(Iterable<E> items,Predicate<E> predicate) {
List<E> resultItems = new
ArrayList<E>();
for(E item :items) {
if(predicate.evaluate(item)) {
resultItems.add(item);
}
}
return resultItems;
}
Now if I can only find a good site to post the finished library once I’m done.