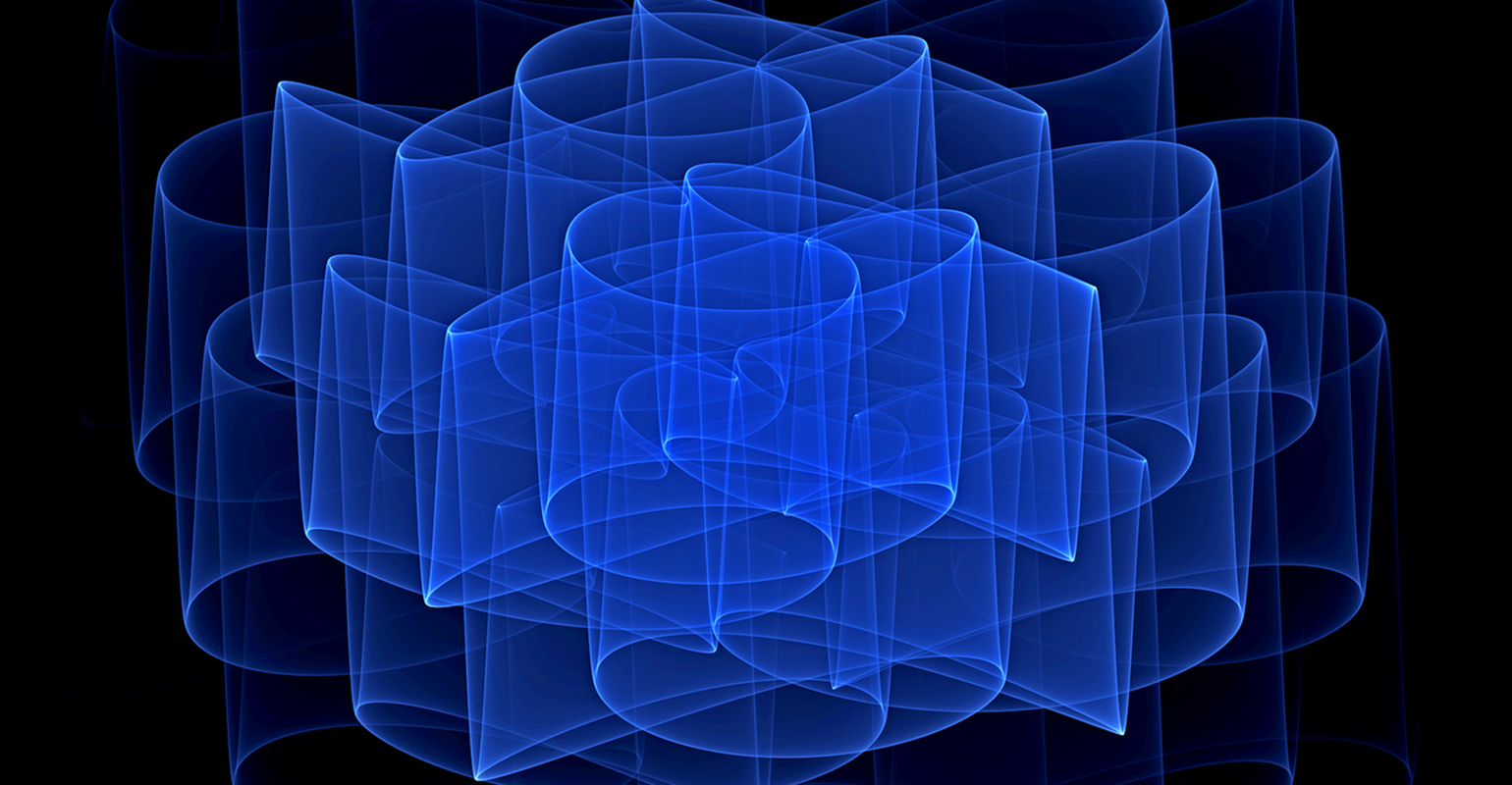
CompositeWPF offers modularity by making it possible to load modules from disk on demand or loading a set modules at startup of the application. One thing that I was missing with this was the possibility lookup the status of modules and which modules are actually available to the application at the moment.
To solve this I wrote a new extension on CompositeWPF that offers the possibility to query a Module status service for a list of available modules and the status of each module.
Usage
To use the module status service you need to add some custom logic to you UnityBootStrapper instance. (Sorry folks, no support for other IoC containers at this moment yet).
The first step is to register a new unity extension with the unity container. This extension will add a new builder strategy to the container. This new builder strategy is placed in the very last stage of object construction and registers the type of the constructed object as loaded module when the constructed type is an instance of IModule.
The next step is registering a singleton instance of the Module Status Service so that the application can use this service to retrieve status information about modules. A sample on how to configure the container for the module status service is shown below:
///
/// Configures the container.
///
protected override void ConfigureContainer()
{
base.ConfigureContainer();
Container.AddExtension(new ModuleTrackingUnityExtension());
Container.RegisterInstance<IModuleStatusService>(Container.Resolve<ModuleStatusService>());
}
After you have registered the module status service with the required container extension you can module status information by invoking the following code:
IModuleStatusService service = Container.Resolve<IModuleStatusService>();
ReadOnlyCollection modules = service.GetModules();
How does it work
The solution is composed of two parts, one is the module status service which is used by the application to retrieve module information. The other part is the module tracking extension for unity.
The Module status service
The module status service is pretty straight forward, it has an internal list to keep track of loaded modules and two methods. One method to register a loaded module and a method to retrieve a list of modules.
The LoadModules method is interesting as it makes use of some internals of the CompositeWPF framework. One of the things CompositeWPF does when you use the UnityBootstrapper, is registering the module enumerator that was supplied in the container. This module enumerator is now available to other components in the application.
You can use this module enumerator in the module status service to retrieve a list of modules that you can expect to be available in the application.
Retrieving a list of modules is quite easy using this technique, but you can’t really see if the module has been actually activated if it is in the list of modules retrieved from the module enumator. For this you need the module tracking extension.
Module tracking extension
The module tracking extension for unity contains some magic to keep track of modules that are being loaded. There are actually two things that happen here:
1. A new builder strategy is added to the object builder chain
The new builder strategy checks if the type being build is a module and registers the type of the object being constructed if it is indeed a module. This builder strategy is added as the very last strategy in the chain, this ensures that all construction logic is performed with success and the module is guaranteed to be activated.
2. The RegisteringInstance event is bound
Although not very likely it is possible to register module instances with the unity container using the RegisterInstance method. For 100% coverage of all possible module activation paths, the RegisteringInstance event is wired up.
Conclusion
While you still have to create your own view to display the module information, this service should provide you with the required information to display module status information and make the task of retrieving module status information a whole lot easier.
The extension can be found on CodePlex in the sourcecontrol of CompositeWPFContrib