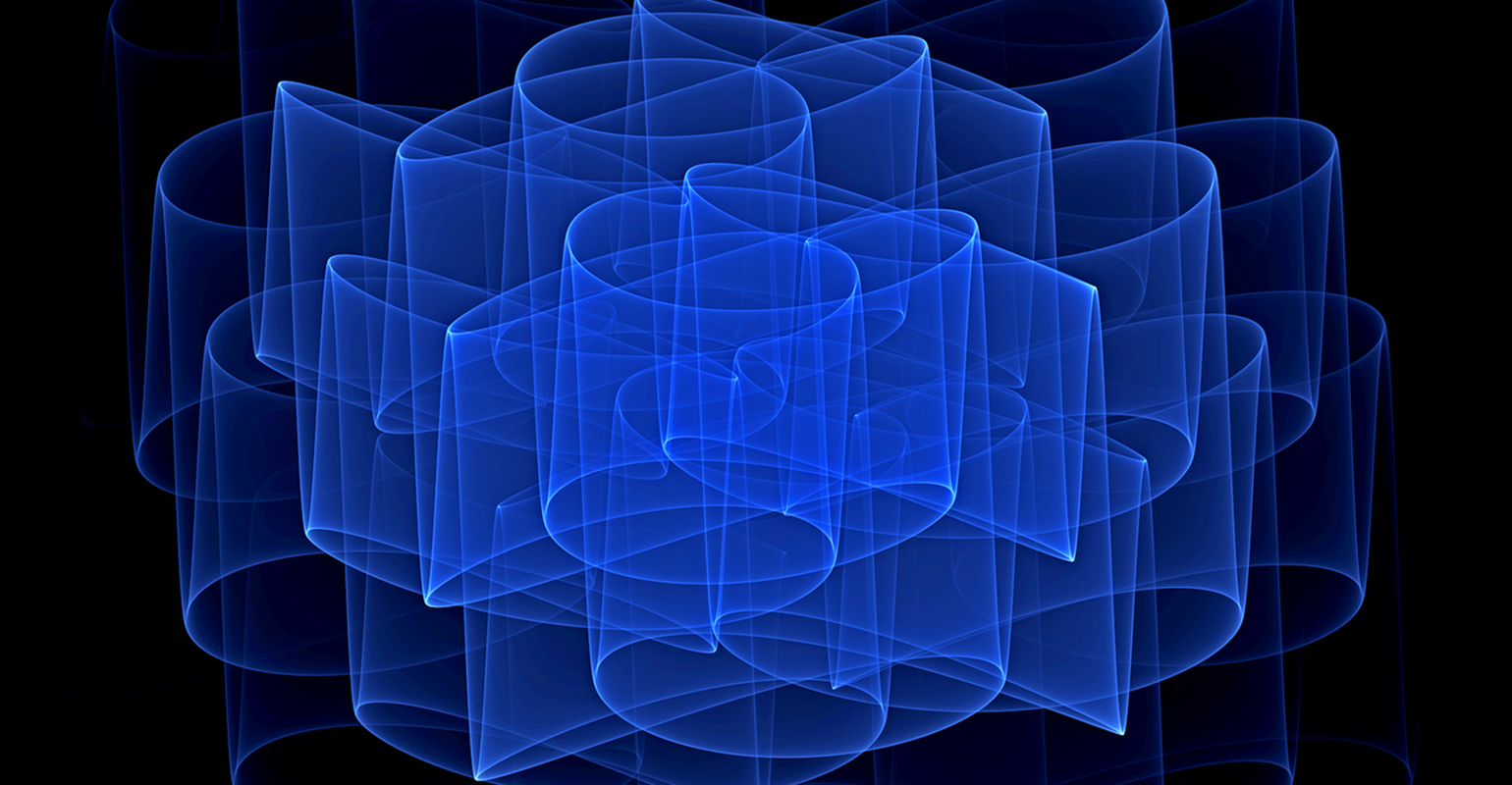
One of the cooler features in Silverlight 4 is the PathListBox control. In this post I will be showing you what the control does using a simple sample application that data binds a set of items to a PathListBox to form a different kind of graph.
What is the PathListBox
The PathListBox control is a list box with a difference. Instead of a normal items panel it uses a path to layout items that are added to the items collection of the control. This allows for a wide range of different scenarios, like the one shown in the screenshot above.
Using the PathListBox
To use the PathListBox control, you need to add a reference to the following assembly:
· Microsoft.Expression.Controls.dll
After you’ve added the reference to the assembly you will be able to use the PathListBox in your application, by adding a new instance of the control to a screen.
1: <Grid x:Name="LayoutRoot" Background="White">
2: <Ellipse x:Name="Circle" HorizontalAlignment="Center" VerticalAlignment="Center" Width="100" Height="100">
3: </Ellipse>
4: <ec:PathListBox ItemsSource="{Binding Measurements}">
5: <ec:PathListBox.ItemTemplate>
6: <DataTemplate>
7: <!-- Data template contents -->
8: </DataTemplate>
9: </ec:PathListBox.ItemTemplate>
10: <ec:PathListBox.LayoutPaths>
11: <ec:LayoutPath SourceElement="{Binding ElementName=Circle}" Orientation="OrientToPath"/>
12: </ec:PathListBox.LayoutPaths>
13: </ec:PathListBox>
14: </Grid>
The most interesting difference compared to a normal ListBox control is the LayoutPaths property that is available on the control. You can provide multiple layout paths to the listbox, along which the items are distributed.
By adding a LayoutPath to the PathListBox, you tell the list box a number of things. The first property “SourceElement” uses a Binding expression to link an existing path. You can use any path type here, for example: Ellipse, Rectangle or a Path. The second property “Orientation” tells the listbox to align the items in the list box along the path, or leave them as they are. These are the most important properties you will be using when working with a single path. The PathListBox control supports multiple layout paths, but I will leave that out for now.
For a full list of the available properties: MSDN Documentation
The PathListBox has the usual ItemsSource and Items collection, so you can either databind a collection of items to it or use the items collection to add content manually.
In the sample, included with this post, you will find that I’ve used data-binding and a custom item template to produce the effect in the screenshot at the beginning of this post. Needless to say you can do some pretty cool things to this control.
The internals of the PathListBox control
The PathListBox control is one of those controls that need a little more inspection, so you get a better idea of how it works and why it works the way it does.
As any good items control, the PathListBox control is made out of several components. The outside of the control is the PathListBox itself. The control template however reveals a few more bits. One of those bits is the PathPanel control. This control is used in combination with the LayoutPath to produce the general layout of the control.
The PathPanel can also be used for situations where you don’t need selection, but it doesn’t support the orientation of items along the associated path. You will have to implement that yourself.
Each item on the PathListBox is contained with a PathListBoxItem control that applies the required render transform to orientate the item along the path specified on the list box. By default it applies the correct rotation and offset. You can however manipulate this as the properties for the orientation and offset are available on the PathListBoxItem, so you can use them within a custom control template if you want to.
Conclusion
Yes, it is powerful and yes you can do a lot of things with it. There is however one tiny problem with the PathListBox control. It would have been much cooler if you were able to use the PathPanel in combination with items that orientate along the path specified on the PathPanel. It does make sense if you look at the rest of the control, but it leaves room for improvement.
Also there are is a small issue with the control template for the PathListBoxItem. There is no way you can specify the RenderTransformOrigin property, which is essential when you want to do stuff like the things I’ve done in the sample.