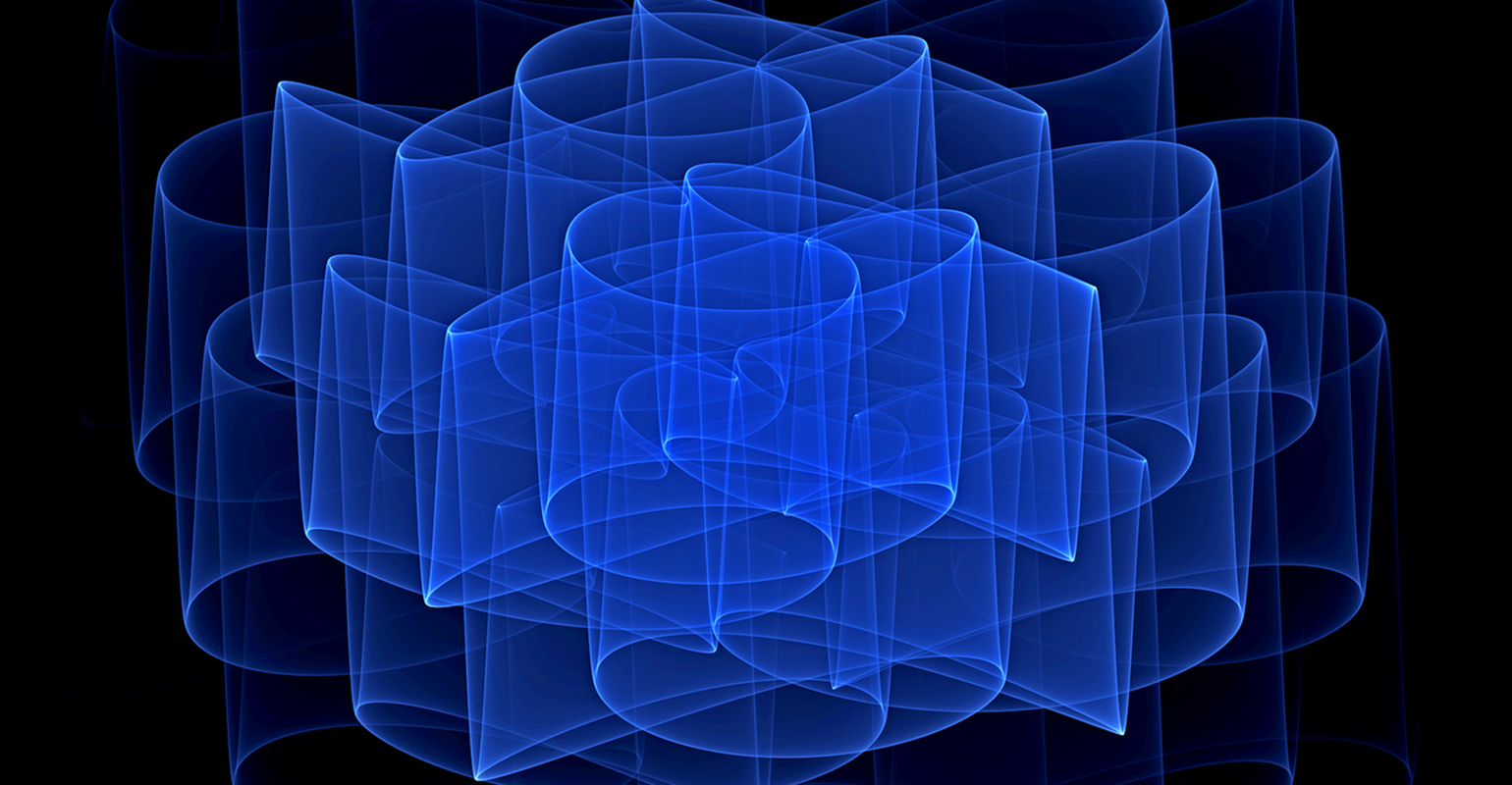
Every year Xamarin hosts his glorious conference named Xamarin Evolve. This year I had the amazing chance to be there in Orlando. Thank you Info Support!
Like every year Xamarin presents the keynote which everyone is looking forward to. During the keynote new features will be presented to the crowd. I was very excited prior to the keynote and mainly because I was hoping for some amazing new Xamarin.Forms features, since I’m a Forms fanatic. In this blogpost you will find an in-depth description of the new features including a wrap up with my personal insights.
Themes
The addition of themes in Xamarin.Forms will make our life as a developer much easier when developing beautiful user interfaces in Forms. As a developer I noticed that I’ve written the same styling for buttons numerous times. Whenever I wanted to modify the styling of a button this meant I had to do this for all other buttons too. Luckily we do not have to do this anymore since Xamarin released their new theming feature.
The new theming feature of Forms looks a little like CSS(defined in XAML) and enables developers to form complex hierarchies that provide a rich theming experience.
The code below describes what it takes to add styling to particular UI elements in Forms. It’s simple and neat syntax.
<StackLayout>
<BoxView StyleClass="HorizontalRule" />
<BoxView StyleClass="Circle" />
<BoxView StyleClass="Rounded" />
</StackLayout>
As you can see it only takes one property, StyleClass, to define a new styling to that particular element. Amazing.
The big question though (I feel your brains cracking) is : How do we define the StyleClass? Defining the style class is pretty easy and the code shown below is pretty self explanatory.
<ResourceDictionary> <!-- DEFINE ANY CONSTANTS --> <Color x:Key="SeparatorLineColor">#CCCCCC</Color> <Color x:Key="iOSDefaultTintColor">#007aff</Color> <Color x:Key="AndroidDefaultColor">#1FAECE</Color> <OnPlatform x:TypeArguments="Color" x:Key="AccentColor" Android="{ StaticResource AndroidDefaultColor }" iOS="{ StaticResource iOSDefaultTintColor }" /> <!-- BOXVIEW CLASSES --> <Style TargetType="BoxView" Class="HorizontalRule"> <Setter Property="BackgroundColor" Value="{ StaticResource SeparatorLineColor }" /> <Setter Property="HeightRequest" Value="1" /> </Style> </ResourceDictionary>
In the snippet above you can see that it takes a ResourceDictionary to define new styles. It’s possible to define Color constants which can be used across the rest of the application. Besides defining constants it’s also possible to style a particular type of element, for example a BoxView. The only thing you have to do is setting the particular property as shown in the snippet.
The new features as described takes us far, but often we want some particular effects on elements that we can’t develop in XAML. For example a rounded corner on the BoxView. For these type of modifications Xamarin came up with ThemeEffects.
DataPages
Besides Themes, Xamarin also announced a new featured called DataPages. Apparently a lot of Xamarin developers needed an easy solution for retrieving and showing data from an existing datasource. DataPages is a solution that should be the answer for this demand.
As a developer the only thing you need to do is: select a datasource, enter your cloud credentials, bind your data and click build. DataPages not only takes care of connecting to the DataSource, it also creates master-detail pages for visualizing the data automatically, without any additional configuration. The following datasources are currently shipped within the preview build:
- JsonDataSource
- AzureDataSource (separate Nuget)
- AzureEasyTableDataSource (separate Nuget)
The first step you have to take is adding your custom theme to the App.xaml like shown below.
<Application >="http://xamarin.com/schemas/2014/forms"
>="http://schemas.microsoft.com/winfx/2009/xaml"
>="clr-namespace:Xamarin.Forms.Themes;assembly=Xamarin.Forms.Theme.Light"
x:Class="DataPagesDemo.App">
<Application.Resources>
<ResourceDictionary MergedWith="mytheme:StandardThemeResources" />
</Application.Resources>
</Application>
The second thing you have to do is create a custom XAML Page of type ListDataPage instead of ContentPage (example below).
public partial class SessionDataPage : Xamarin.Forms.Pages.ListDataPage // was ContentPage
{
public SessionDataPage ()
{
InitializeComponent ();
}
}
The last step to get DataPages working is by adding a DataSource to the page (example below).
<?xml version="1.0" encoding="UTF-8"?>
<p:ListDataPage >="http://xamarin.com/schemas/2014/forms"
>="http://schemas.microsoft.com/winfx/2009/xaml"
>="clr-namespace:Xamarin.Forms.Pages;assembly=Xamarin.Forms.Pages"
x:Class="DataPagesDemo.SessionDataPage"
Title="Sessions" StyleClass="Events">
<p:ListDataPage.DataSource>
<p:JsonDataSource Source="http://demo3143189.mockable.io/sessions" />
</p:ListDataPage.DataSource>
</p:ListDataPage>
As you can see the XAML also contains StyleClass=”Events” which is required for using DataPages. The Events style has styles that match the datasource (eg. “title”, “image”, “presenter”).It’s required to have a style (custom theme), which make this all a little complex at the moment.
Native Embedding
Since the release of Forms everybody was stating that it’s quite difficult to do some custom UI. The next releases brought us renderers which made our life a little easier and allowed us to incorporate more specific and complex UI elements.
With the release of Native Embedding it should be easier to insert native controls into a Xamarin.Forms UI. The way it works is pretty, but it requires the use of shared projects: making it impossible for applications using PCL’s.
I actually tried this out myself and it was pretty easy setting up a shared project and adding a native control (UILabel) in a Xamarin.Forms layout, as seen in the code snippet below.
using System; #if __IOS__ using UIKit; #endif using Xamarin.Forms; using Xamarin.Forms.Platform.iOS; namespace testProject { public class App : Application { public App() { var testPage = new ContentPage(); var newStackLayout = new StackLayout() { Padding = new Thickness() { Bottom = 0, Left = 0, Right = 0, Top = 20 } }; #if __IOS__ var uiLabel = new UILabel { MinimumFontSize = 14f, Lines = 0, LineBreakMode = UILineBreakMode.WordWrap, Text = "Check blogs.infosupport.com for more information :-)", }; newStackLayout.Children.Add(uiLabel); #endif testPage.Content = newStackLayout; MainPage = testPage; } } }
As you can see it is pretty easy to incorporate native controls in an existing Xamarin.Forms interface. I actually have a strong opinion on this, which can be found in the wrap-up.
Xamarin.Forms Previewer
This feature is something I really love and that’s the reason why I saved it for last. I honestly think this is revolutionary in the mobile world. The Xamarin.Forms previewer allows you to write XAML code and see the changes immediately on screen. This means we do not have to deploy our code anymore every time we make a small modification. This allows us to work a lot faster when developing complex screens with Xamarin.Forms.
As you can see in the example above it works pretty well! It renders the UI as you type, which is neat. It’s also possible to select a specific platform (iOS/Android), type of device and orientation. The previewer actually works with XAML and renderers, but sadly not with pages built in code. So, XAML only, at least for now..
Wrap up
I think Xamarin made some awesome features for Forms, especially the Previewer which is simply amazing. I tried out to get the themes working, but failed due to required NuGet packages that waren’t available yet at the time of writing. I honestly think that theming could be an incredible feature, but from what I have seen now it is pretty hard to develop a custom theme.
Regarding DataPages, for now, I can’t see a direct benefit. The use of DataPages require you to actually make a custom theme in order to show the data in the view (which is hard). Of course you are avoiding all the hassle of retrieving data, but this also means you’re losing control of retrieving this data.
Native Embedding is pretty slick, but the downside is that it only works for Shared projects. If you are a fan of using shared projects, like Miguel de Icaza, you’re all set. If you rather like to use PCL’s (like me): native embedding is not for you. It’s currently not possible to use native embedding in PCL’s, because of the abscence of native API’s like UIKit.
Xamarin Previewer is simply amazing, but it’s currently not mature enough to detect some scenarios. I noticed that the previewer doesn’t show a navigationbar when the page is wrapped in a NavigationPage. I also noticed it isn’t possible to use ContentPages with a custom base class.
Evolve was an amazing event, with amazing people and amazing new features. I think the acquisition of Xamarin by Microsoft can be a good thing and I’m honestly looking forward to the future of mobile development with Xamarin.
Source: blog
2 comments
Nice write-up Mittch! Re: Native Embedding… You could always do native embedding while using PCL with a little bit of DI. Have the shared UI reach into the native one via one interface (via DI or something) and you can pass the parent layout as a parameter to add the native controls to. iOS knows about Forms, just not the other way around…
Roy Cornelissen
Yikes! Why didn’t I see that? You’re totally right 🙂 Thanks for the addition!
Mittchel van Vliet